
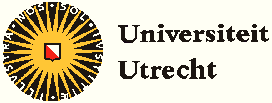
INFOMGPGame Physics
Assignment
Objective: To implement basic and advanced concepts using a 3D physics engine (Bullet Physics Library).
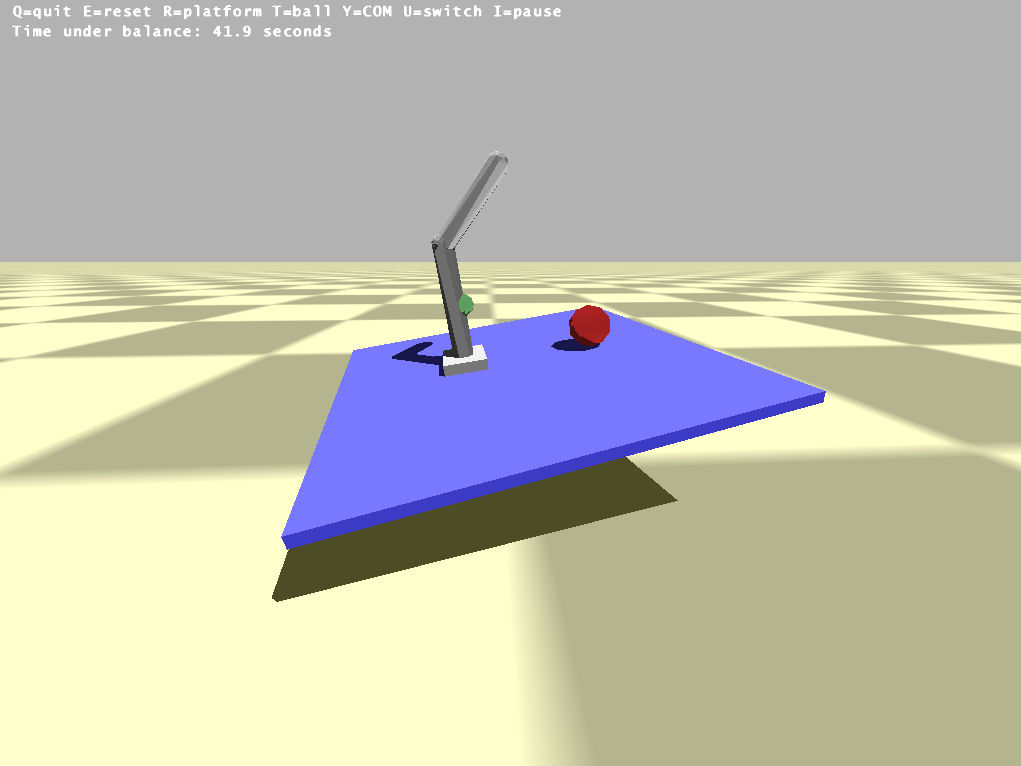
Description: The practical assignment has to be done in groups of 5 or 6 students. It is mostly making use of resources from the lectures and from literature (and possibly from your comparative essays). The primary task is to design an active physics controller for a virtual creature.
Minimal requirements: The creature is a one-leg animal with 1 foot, 1 ankle joint, 1 lower leg, 1 knee joint and 1 upper leg. The properties of the creature are given, e.g. mass and shape of the body parts, friction coefficients, max torques generated at joint etc. and should not be changed in the minimal requirements task. The creature needs to keep its balance on a mobile platform while balls are thrown at it. The students have to implement PD controllers for emulating dynamic responses to external perturbations and for tracking the balanced pose. The creature has to keep its balance as long as possible on the platform. The controllers are joint-space motion controllers where balance is ensured using the Center of Mass (COM) and Center of Support Polygon (CSP). The resulting error in balance is fed to the PD joint controllers.
To meet the minimal requirements the PD controllers and balance algorithm have to be correctly implemented and demonstrated (using the default environment), and as a result the creature is able to maintain indefinitely its balance on a static platform. On a mobile platform, the creature should be able to keep balance on average at least 90 seconds. With both the mobile platform and the balls on, the creature should keep its balance on average at least 30 seconds. Each degree of freedom of the creature is actuated via a PD controller tracking the correct balance pose. The gains have to be defined to have a reactive tracking of the pose while producing non-jerky motions and being responsive to the external perturbations.The algorithm to update the pose of the creature is as follows:
- Compute the COM in world coordinate system
- Describe the ground projected CSP in world coordinate system
- For each DOF
- Describe the ground projected CSP in DOF-BODY coordinate system
- Describe the ground projected COM in DOF-BODY coordinate system
- Calculate the balance error solvable by a DOF rotation (inverted pendulum model)
- Feed the balance error to the PD DOF controller and apply resulting 'torque' (here angular motor velocity, conversion from error to torque/motor velocity is done by the gains of the PD controller)
To get a higher grade: If implemented, documented and presented correctly, minimal requirements can give up to 4 points. To get a higher grade, you can implement several of the following additional tasks. More stars mean more difficult to implement. To be part of assignment a task has to be fully implemented and documented. In these additional tasks you are allowed to modify the properties of the creature (mass, size, friction etc.).
- Propose a balance controller for a more complex creature, for example with
- More precise collisions (e.g. non-primitive shapes)
- Detachable and breakable limbs (e.g. from max internal torque and impact force)
- Additional degrees of freedom (e.g. a ball-and-socket ankle joint)
- Extra limbs that also move to maintain balance (e.g. under windy conditions)
- Soft bodies that passively react to the motion (e.g tails, hairs or clothes)
- Different balance or falling strategies (e.g. create protective steps or poses)
- Propose a controller for another task, for example
- To dodge or batt the balls
- To compete over an object
- To walk or run or height/length jump
- To fight with an opponent
- Integrate other constraints, for example
- Pose dependent joint limits
- Offline automatic optimization of controllers (e.g. to calculate optimal gains for a specific task)
- Tracking of pre-recorded motions or keyframed poses (e.g. to improve realism of motion)
- Actuation from external forces, virtual forces or muscle forces instead of joint torques
- Add more interactions, for example with
- User controlled forces or torques
- Environmental forces (e.g. push from other creatures, wind field, blast wave from an explosion, fluid resistance, buoyancy)
- Environmental constraints (e.g. staircase, holes, bumps, icy/rough surfaces)
- Soft bodies (e.g. jump on trampoline, walk in mud, swim in water)
- Improve the game physics engine, for example
- Implement another numerical integration method
- Implement another collision detection technique
- Implement another collision solving technique
- Experiment on anything you can imagine, so be creative but realistic!
By May 19, you have to submit by email to the teacher the list of the members of each team. You also have to indicate in that email the tasks you plan to implement. This can of course be only a tentative list. More, different or less tasks can eventually be completed by the end of the course.
Forum: A forum has been created for the course. The students can access it at http://infomgp.boards.net/. You first have to create an account and register to the forum (link at the top left). Then you will have access to its content and the ability to post. The forum is meant to be used (1) to ease the creation of groups; (2) to discuss practical issues that some students might already have encountered and solved (e.g. in Bullet SDK). The forum should not be used to share any code, and it will be monitored. The forum is not meant to ask questions to the lecturer, but I might reply to some posts if it's relevant (and if I have time).
Advice: Start as soon as possible!
Software:
A framework has been prepared for you so that you do not have to care about the creation of the application, compilation and linking: => Download the framework <=
This framework is written in C++. The knowledge of an object-oriented language is a pre-requisite for this course.
The application is build on top of the physics engine Bullet Physics Library. You will find information and tutorials on their website. The user manual can be downloaded here. The SDK documentation (not so many comments unfortunately) can be found here and their forum here.
To edit the source code, open the solution file INFOMGP.sln with Visual Studio 2010 or higher. You will have mainly to edit the files Creature.h, Creature.cpp and most probably to create many others.
Use the key Q to quit, the key E to reset the simulation, the key R to rotate the platform, the key T to throw balls, the key Y to display the COM and the key I to pause. To move the camera press the Ctrl key and move the mouse while holding the Left Button for rotating, the Right Button for zooming and the Middle Button for translating. Executables are created in the solution directory.
Please for the sake of the reviewer, do not create your own solution with other tools (even if a different renderer would produce nicer scenes, or another physics engine would be more stable etc.). You should not need any third-party libraries, but if you have a real strong need that you can argue for, you can ask for my approval. In that case, you will also have to deliver the necessary libraries.
Grading: You can access the detailed grading system that will be used here.
Deliverables: You have to hand in an archive containing:
- A report on the completed tasks (pdf or word)
- The source code build upon the provided template
Mark all deliverables clearly with your names and student numbers. Submit only 1 archive per group. During the submission process (second screen) do not forget to add the SolisIDs and the student numbers of every student in your group. The root of the archive should be a folder with the name: STUDENTNUMBER1 STUDENTNUMBER2 [...]. You have to provide both release and debug executables so I can directly execute the program. Make sure that you have cleaned your submission (no useless temporary files). Please double check that it is working on a fresh machine. The submission deadline is Friday, 27 June 2014 at 18:00. To send your work, you will use the CS-UU submission system available at http://www.cs.uu.nl/docs/submit (select 'Game physics - Practical Assignment'). Please DO NOT send your assignment by email. After a successful submission you should receive a confirmation email in your student email account. If not, please contact me. If your archive is too large for the submit system, you can hand it in directly at my office BBL-420 (you have to arrange a meeting with me).